Ready-to-use CKEditor 5 build with the file manager
The easiest way to install CKEditor 5 with a file manager and an image editor on board is to use a ready build.
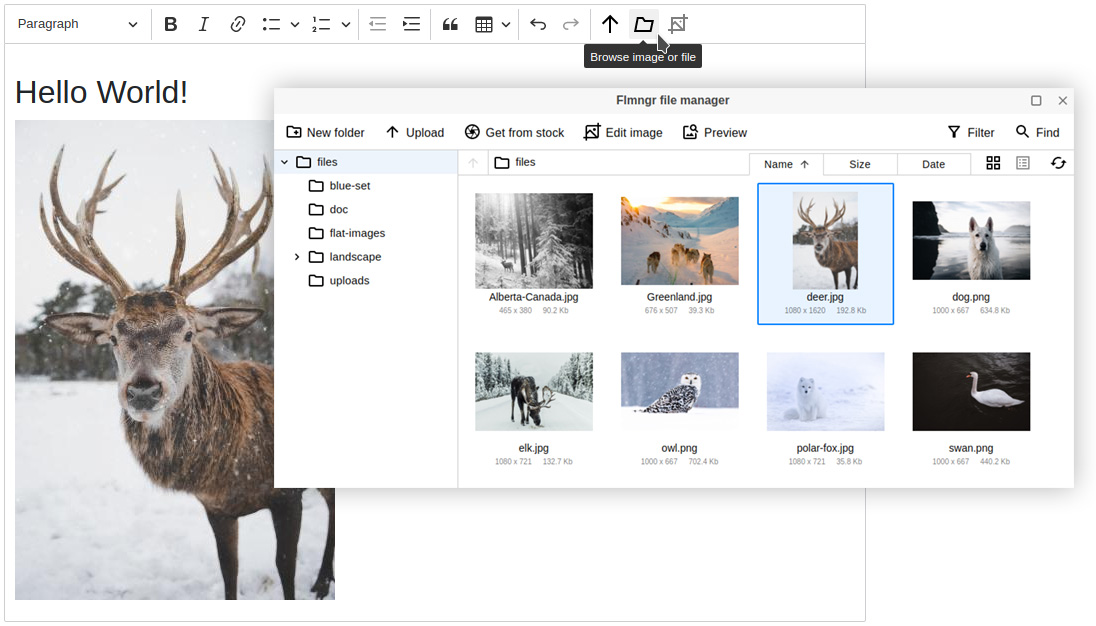
Include JS snippet to HTML page
We have compiled and hosted a build of CKEditor 5 with Flmngr file manager enabled there.
Include this snippet to your page to load CKEditor 5 build from our CDN:
<script src="https://cloud.flmngr.com/v/latest/sdk/ckeditor5-flmngr.js"></script>
Alternatively, you can download this URL to your server and include it directly but automatic updates won't work.
Initialize CKEditor 5
Here is a sample code how to initialize CKEditor 5. Place this script to be loaded after the build:
<script type="text/javascript">
CKEditor5WithFileManager.create(
document.querySelector('#editorId'),
{
Flmngr: {
// Get your API key in the Dashboard: https://flmngr.com/dashboard
// apiKey: "..."
}
}
);
</script>
Install the backend
Follow server installation manual on your server where you would like to store files and images for the file manager.
Be sure that you've specified your API key in the client installation and link it to the server using Dashboard.
Ready to use
Customize
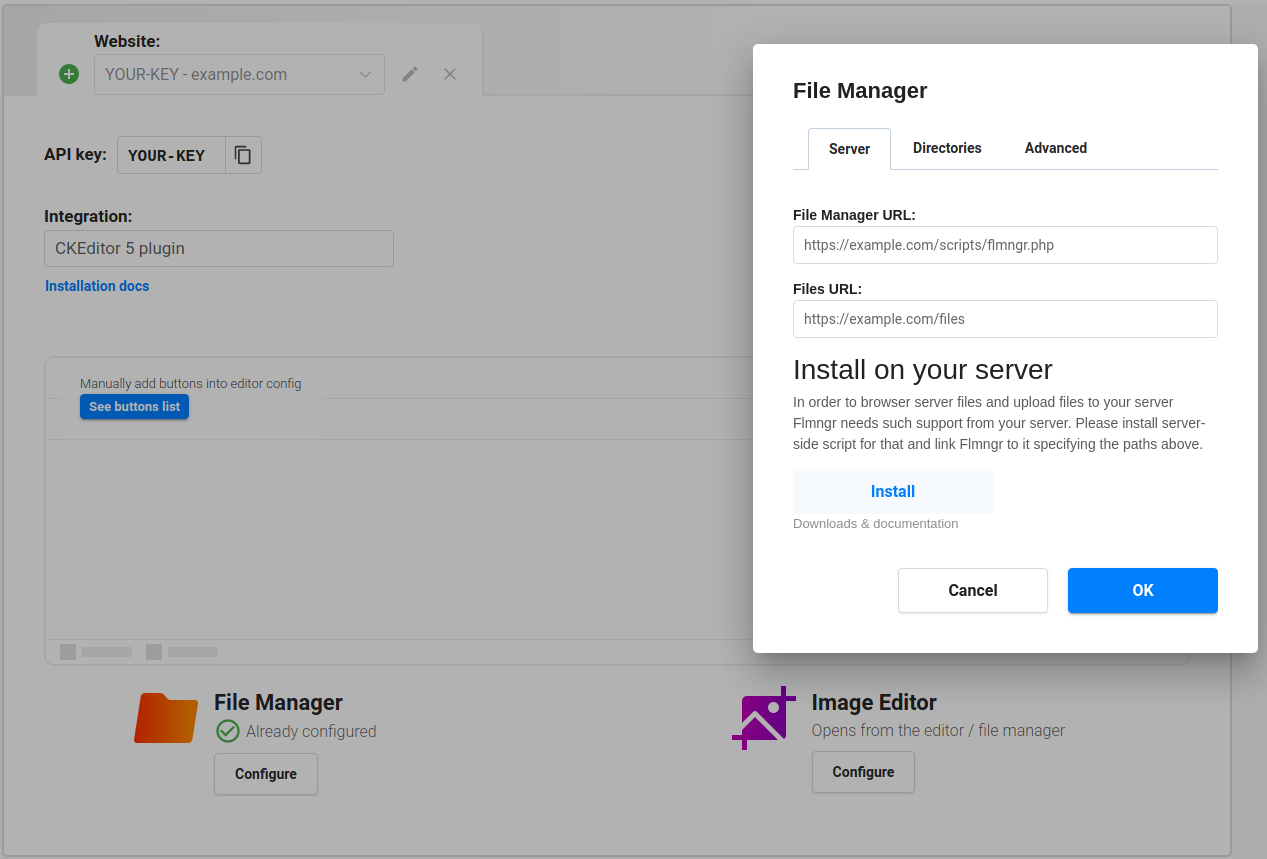
Configure CKEditor 5 file manager visually inside the Dashboard. This configuration can be dynamically overridden by passing API parameters directly.
Samples
There is a sample of CKEditor 5 with the file manager installation and using its API:
Source
Demo: you can fork/clone or just download it and check it on your computer without any installation, just click on the file sample/index.html
. Please use this file as a sample of how to configure CKEditor 5.